omni.kit.window.file_exporter¶
The file_exporter extension provides a standardized dialog for exporting files. It is a wrapper around the FilePickerDialog
,
but with reasonable defaults for common settings, so it’s a higher-level entry point to that interface.
Nevertheless, users will still have the ability to customize some parts but we’ve boiled them down to just the essential ones.
Why you should use this extension:
Present a consistent file export experience across the app.
Customize only the essential parts while inheriting sensible defaults elsewhere.
Reduce boilerplate code.
Inherit future improvements.
Checkpoints fully supported if available on the server.
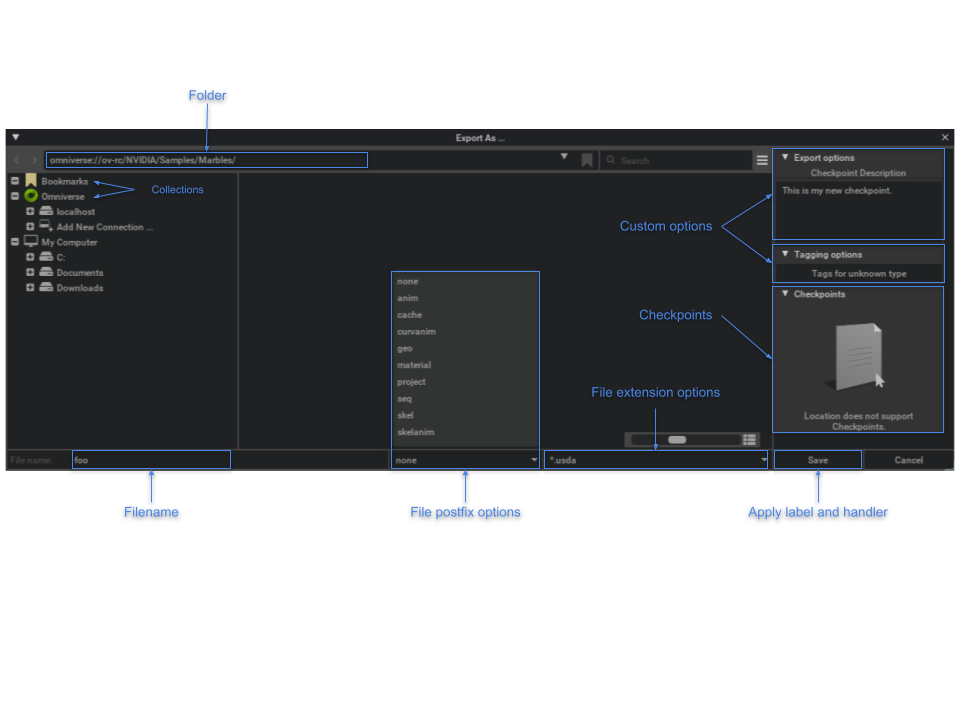
Quickstart¶
You can pop-up a dialog in just 2 steps. First, retrieve the extension.
# Get the singleton extension object, but as weakref to guard against the extension being removed.
file_exporter = get_file_exporter()
if not file_exporter:
return
Then, invoke its show_window method.
file_exporter.show_window(
title="Export As ...",
export_button_label="Save",
export_handler=self.export_handler,
filename_url="omniverse://ov-rc/NVIDIA/Samples/Marbles/foo",
)
Note that the extension is a singleton, meaning there’s only one instance of it throughout the app. Basically, we are assuming that you’d never open more than one instance of the dialog at any one time. The advantage is that we can channel any development through this single extension and all users will inherit the same changes.
Customizing the Dialog¶
You can customize these parts of the dialog.
Title - The title of the dialog.
Collections - Which of these collections, [“bookmarks”, “omniverse”, “my-computer”] to display.
Filename Url - Url to open the dialog with.
Postfix options - List of content labels appended to the filename.
Extension options - List of filename extensions.
Export options - Options to apply during the export process.
Export label - Label for the export button.
Export handler - User provided callback to handle the export process.
Note that these settings are applied when you show the window. Therefore, each time it’s displayed, the dialog can be tailored to the use case.
Filename postfix options¶
Users might want to set up data libraries of just animations, materials, etc. However, one challenge of working in Omniverse is that everything is a USD file. To facilitate this workflow, we suggest adding a postfix to the filename, e.g. “file.animation.usd”. The file bar contains a dropdown that lists the postfix labels. A default list is provided but you can also provide your own.
DEFAULT_FILE_POSTFIX_OPTIONS = [
None,
"anim",
"cache",
"curveanim",
"geo",
"material",
"project",
"seq",
"skel",
"skelanim",
]
A list of file extensions, furthermore, allows the user to specify what flavor of USD to export.
DEFAULT_FILE_EXTENSION_TYPES = [
("*.usd", "Can be Binary or Ascii"),
("*.usda", "Human-readable text format"),
("*.usdc", "Binary format"),
]
When the user selects a combination of postfix and extension types, the file view will filter out all other file types, leaving only the matching ones.
Export options¶
A common need is to provide user options for the export process. You create the widget for accepting those inputs,
then add it to the details pane of the dialog. Do this by subclassing from ExportOptionsDelegate
and overriding the methods, ExportOptionsDelegate._build_ui_impl()
and (optionally) ExportOptionsDelegate._destroy_impl()
.
class MyExportOptionsDelegate(ExportOptionsDelegate):
def __init__(self):
super().__init__(build_fn=self._build_ui_impl, destroy_fn=self._destroy_impl)
self._widget = None
def _build_ui_impl(self):
self._widget = ui.Frame()
with self._widget:
with ui.VStack(style={"background_color": 0xFF23211F}):
ui.Label("Checkpoint Description", alignment=ui.Alignment.CENTER)
ui.Separator(height=5)
model = ui.StringField(multiline=True, height=80).model
model.set_value("This is my new checkpoint.")
def _destroy_impl(self, _):
if self._widget:
self._widget.destroy()
self._widget = None
Then provide the controller to the file picker for display.
self._export_options = MyExportOptionsDelegate()
file_exporter.add_export_options_frame("Export Options", self._export_options)
Export handler¶
Pprovide a handler for when the Export button is clicked. In additon to filename
and dirname
, the handler
should expect a list of selections
made from the UI.
def export_handler(self, filename: str, dirname: str, extension: str = "", selections: List[str] = []):
# NOTE: Get user inputs from self._export_options, if needed.
print(f"> Export As '{filename}{extension}' to '{dirname}' with additional selections '{selections}'")
Demo app¶
A complete demo, that includes the code snippets above, is included with this extension at “scripts/demo_file_exporter.py”.
-
class
omni.kit.window.file_exporter.
FileExporterExtension
¶ A Standardized file export dialog.
-
add_export_options_frame
(name: str, delegate: omni.kit.window.filepicker.detail_view.DetailFrameController)¶ Adds a set of export options to the dialog. Should be called after
show_window()
.- Parameters
name (str) – Title of the options box.
delegate (
ExportOptionsDelegate
) – Subclasses specified delegate and overrides_build_ui_impl()
to provide a custom widget for getting user input.
-
click_apply
(filename_url: Optional[str] = None)¶ Helper function to progammatically execute the apply callback. Useful in unittests
-
click_cancel
()¶ Helper function to progammatically execute the cancel callback. Useful in unittests
-
hide_window
()¶ Hides and destroys the dialog window.
-
show_window
(title: Optional[str] = None, width: int = 1080, height: int = 450, show_only_collections: Optional[List[str]] = None, file_postfix_options: Optional[List[str]] = None, file_extension_types: Optional[List[Tuple[str, str]]] = None, export_button_label: str = 'Export', export_handler: Optional[Callable[[str, str, List[str]], None]] = None, filename_url: Optional[str] = None)¶ Displays the export dialog with the specified settings.
- Keyword Arguments
title (str) – The name of the dialog
width (int) – Width of the window (Default=1250)
height (int) – Height of the window (Default=450)
show_only_collections (List[str]) – Which of these collections, [“bookmarks”, “omniverse”, “my-computer”] to display.
file_postfix_options (List[str]) – A list of filename postfix options. Nvidia defaults.
file_extension_types (List[Tuple[str, str]]) – A list of filename extension options. Each list element is a (extension name, description) pair, e.g. (“.usdc”, “Binary format”). Nvidia defaults.
export_button_label (str) – Label for the export button (Default=”Export”)
export_handler (Callable) – The callback to handle the export. Function signature is export_handler(filename: str, dirname: str, postfix: str, ext: str, selections: List[str]) -> None
filename_url (str) – Url of the target file, excluding filename extension.
-
-
omni.kit.window.file_exporter.
get_file_exporter
() → omni.kit.window.file_exporter.extension.FileExporterExtension¶ Returns the singleton file_exporter extension.